A simple introduction to MVVM
A simple introduction to MVVM
The Model-View-ViewModel pattern, or for short MVVM, is the state-of-the-art pattern for WPF applications.
The MVVM pattern attempts to gain both advantages of separation of functional development provided by MVC, while leveraging the advantages of data-bindings and the framework by binding data as close to the pure application model as possible. It uses the binder, ViewModel, and any business layers data checking features to validate incoming data.
To give an illustration of what I mean, let's look at the case of this graphic below :
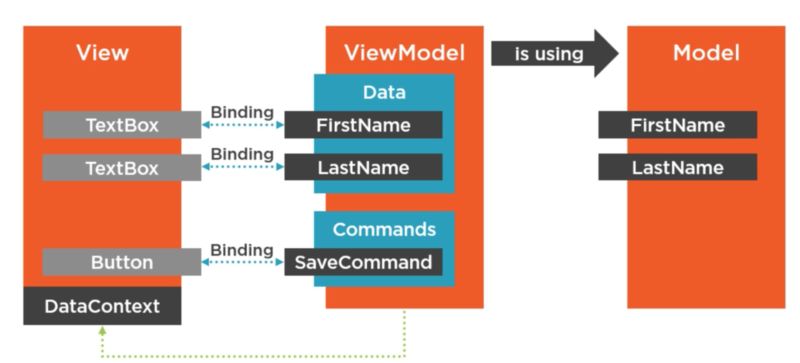
The Model : which can be for example a Friend class with the properties FirstName, and LastName. Then you have a ViewModel, which is another class that is using the Model.
The ViewModel : has public properties FirstName, and LastName that contains data, that are accessing the same properties of the Friend class which is our Model. All the public properties in the ViewModel are defined exactly how they are required by the View.
The View : is accessing those properties by using data bindings.
So for example we have a FisrtName and LastName textboxes in the View, so both textboxes should be bound to the properties of the same name of the ViewModel. To make this data binding work, the ViewModel should be assigned to the DataContext property of the View.
Another key thing to remember is that you don't only have data properties, but also some actions that happen on the View. These actions are exposed by the ViewModel in the form of Commands. So besides the public properties with data, the ViewModel has public properties of type ICommand.
For instance, the View can have a save button which it's using the SaveCommand property of the ViewModel with a data binding.
Now one thing that I want to mention here, is when you look at the graphic, you see that the ViewModel is independent from the View. The ViewModel only knows the Model but it does not know the View. And this leads to many advantages of the the MVVM pattern.
The Advantages of the MVVM pattern
Building your application using the MVVM pattern gives you some advantages among them :
Maintainability
The View logic implmeneted in the ViewModel is separated from the View, when we build the View we don't have to care about the logic. For example the view binds to a SaveCommand property of the ViewModel, the View has just to take care of the databinding, how the SaveCommand works and how is it implemented is part of the ViewModel.
This separation keeps the application simple to maintain.
Testability
Another great advantage of MVVM is testability. As the ViewModel is completely UI-independent, it's really great to be unit-tested. In the ViewModel, you don't have any code with controls, layout, and other view related stuff. You have just the class with public properties and logic. So this makes it very good to perform unit testing.
Thanks for reading!